Camel Case vs. Snake Case vs. Pascal Case — Naming Conventions
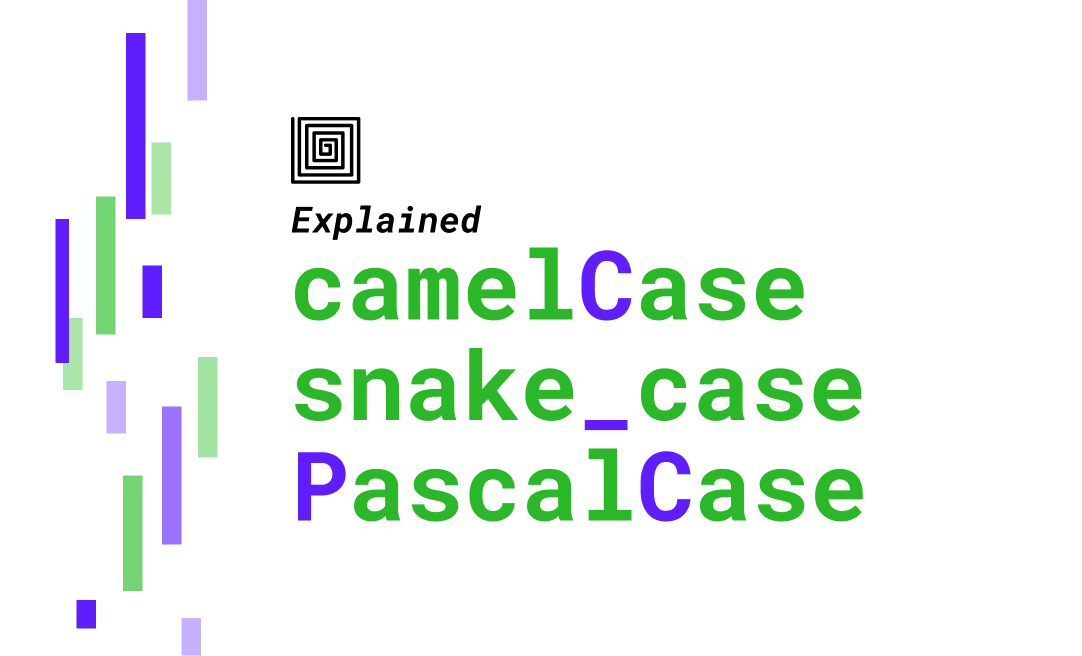
Naming conventions based on language
The most common naming conventions
Camel case usage in TypeScript
Snake case usage in TypeScript
Pascal case usage in TypeScript
Comparison of naming conventions in other programming languages
The tldr
Naming conventions dictate the way you write names (for methods, folders, variables and so on). The three most common are camel case, pascal case, and snake case.
- Camel case always starts out lowercase with each word delimited by a capital letter (like personOne, textUtil, thingsToDo)
- Pascal case is similar to camel case, but the first letter is always capitalized (like PersonOne, TextUtil, ThingsToDo)
- Snake case means that we delimit words with an underscore (like person_one, text_util, things_to_do)
Continue reading for more information on usage best practices.
Introduction
There are many different ways to improve the maintainability of your codebase. One of the first things developers learn how to do is to decide upon and enforce a set of coding conventions to create consistency in the formatting and style of your code. Under the umbrella of coding conventions exists the idea of naming conventions.
Camel case, snake case, and pascal case are three of the most common types of programming language naming conventions found across all programming languages.
In this article, we discuss why naming conventions matter; we look at camel case, snake case, and pascal case — and for each, we demonstrate with examples and learn when to use each in a variety of programming languages.
Naming conventions
What are they?
Naming conventions are rules that dictate the way you name the various files, folders, and tokens in our code (such as variables, functions, classes, methods, objects, and so on).
Naming conventions based on language
While a team can decide upon naming conventions for any project, each programming language has standard naming conventions for different tokens.
For example, in C-styled languages like Java, JavaScript, and TypeScript, while it's conventional to use the camel case convention for variables, functions, method names, and object properties, it's common practice to use the pascal case convention for classes, interfaces, and namespaces. That's not to say that you can't use snake case or other unconventional naming conventions for a language like Java or JavaScript. In most cases, your code will still compile and run, but it's good to generally try to avoid going against the typical conventional naming practices for the language. Because there are usual language conventions, adhering to those rules can help improve the ease of future maintenance.
Why naming conventions?
The entire idea behind naming conventions is to help make code more maintainable for the reader. By enforcing a convention and naming tokens consistently, we accomplish two things: discoverability and understanding.
- Discoverability: How quickly can someone find a folder, file, feature, class, or method they need to change?
- Understanding: How fast can someone look at our code and understand what it is they're looking at?
We want to make it very easy for developers on our team to find what they're looking for, understand what it is, and understand how to use it as quickly as possible.
The most common naming conventions
Across all programming languages, the most common naming conventions are the following:
- Camel case (ex: someVariable)
- Snake case (ex: some_variable)
- Pascal case (ex: SomeVariable)
Let's explore each one.
Camel case
Camel case is perhaps the most common naming convention of all three. A camel case word is a single or compound word that uses capital letters to make different parts of the word easier to read.
It adheres to the following rules:
- The word doesn't end on a capital letter (like someVariablE)
- Capital letters can only appear at the start of the second word (like someVariable).
- No dots, underscores, numbers, dashes, or any other special characters are allowed within the word (like som.eVar-1able)
In most C-style languages, we use camel case to denote variables, properties, attributes, methods, and functions.
Some make a distinction between lowerCaseCamel and UpperCaseCamel, but most developers refer to the former as merely camel case and the latter as pascal case (which we'll talk about later).
Camel case examples
- someVariable
- eventDispatcher
- eventsRepo
- slackService
- parentComment
Camel case usage in TypeScript
In TypeScript & JavaScript, the camel case convention is used to signify that a token is a variable, function, method, parameter, or property.
type UserProps = {
firstName: string; // Used to signify properties
lastName: string;
email: string
}
interface Serializable {
toJSON (): string; // A method also camel cased
}
class User implements Serializable {
private props: UserProps; // Camel cased property
// constructor and parameters are camel cased
constructor (firstName: string, lastName: string) {
this.props = { firstName, lastName };
}
public toJSON (): string {
return JSON.stringify(this.props);
}
}
// Variables are camel case
const userOne = new User('Khalil', 'Stemmler');
const userTwo = new User('Nick', 'Cave');
Snake case
Snake case (also referred to as underscore case) is when all the letters of the word are lower case but delimited by an underscore.
We seldom use the snake case coding convention in C-style languages like Java, JavaScript, and TypeScript. They're typically only used in programming languages like these to denote constants. To denote a constant, we typically implement screaming snake case: snake case with all of the letters capitalized.
You'll most commonly see snake case in the Python scripting language as is popularized in the Python style guide.
Snake case examples
- some_variable
- event_dispatcher
- events_repo
- slack_service
- parent_comment
Snake case usage in TypeScript
Let’s look at the same TypeScript example and amend it ever-so-slightly to demonstrate when we may utilize the snake case naming convention.
type UserProps = {
firstName: string;
lastName: string;
email: string
}
interface Serializable {
toJSON (): string;
}
class User implements Serializable {
private props: UserProps;
private static MAX_USERS: number = 10; // Screaming snake case // to depict a constant
constructor (firstName: string, lastName: string) {
this.props = { firstName, lastName };
}
public toJSON (): string {
return JSON.stringify(this.props);
}
}
// Variables are camel case
const userOne = new User('Khalil', 'Stemmler');
const userTwo = new User('Nick', 'Cave');
As you can see, snake case isn't a prevalent naming convention in C-styled languages like TypeScript. We only tend to use it when we're declaring constants.
Snake case usage in Python
In Python, however, the snake case naming convention is much more common. Taking the same TypeScript example translated to Python, look at the widespread usage of snake case.
import json
class User:
props = {
"first_name": "", # Properties of dictionary objects
"last_name": ""
}
MAX_USERS = 10 # Screaming snake case on constants
# Used on method parameters
def __init__(self, first_name, last_name):
self.props["first_name"] = first_name
self.props["last_name"] = last_name
# Usage on method names
def to_JSON(self):
return json.dumps(self.props)
# We even use snake case to for naming variables
user_one = User("Khalil", "Stemmler")
user_two = User("Nick", "Cave")
Pascal case
Pascal case follows the same camel case naming convention rules — all but one: we capitalize the first letter (e.g., SomeVariable).
In object-oriented languages like Java and TypeScript, we use pascal case to denote classes, namespaces, and abstractions like interfaces.
In scripting languages like Python, you'll rarely find camel or pascal case code except when defining a class's name.
Pascal case examples
- SomeVariable
- EventDispatcher
- EventsRepo
- SlackService
- ParentComment
Pascal case usage in TypeScript
Continuing with the same example, let’s look at where pascal case is used in TypeScript code.
type UserProps = { // Types are also abstractions
firstName: string;
lastName: string;
email: string
}
interface Serializable { // Interfaces use pascal case
toJSON (): string;
}
class User implements Serializable { // Class names use pascal case
private props: UserProps;
private static MAX_USERS: number = 10;
constructor (firstName: string, lastName: string) {
this.props = { firstName, lastName };
}
public toJSON (): string {
return JSON.stringify(this.props);
}
}
const userOne = new User('Khalil', 'Stemmler');
const userTwo = new User('Nick', 'Cave');
Comparison of naming conventions in other programming languages
Here’s a table that depicts all of the typical naming conventions for the languages: Python, C#, TypeScript, and Java.
Python | C# | TypeScript | Java | |
---|---|---|---|---|
functions & methods | snake_case() | PascalCase() | camelCase() | camelCase() |
classes | PascalCase | PascalCase | PascalCase | PascalCase |
interfaces | N/A | PascalCase | PascalCase | PascalCase |
namespaces | N/A | PascalCase | PascalCase | PascalCase |
constants | snake_case | SCREAMINGSNAKE\CASE | SCREAMING_SNAKE_CASE | SCREAMING_SNAKE_CASE |
Conclusion
In summary,
- Coding conventions are patterns, techniques, styles, and approaches to improve the maintainability of your code, and naming conventions are one of such techniques.
- Naming conventions improve maintainability by using consistency to optimize for discoverability and understanding.
- Naming conventions depend on the language. Each language has its own naming conventions that specify the use of programming tokens.
- Camel case (ex: camelCase) is usually for variables, properties, attributes, methods, and functions.
- Snake case (ex: snake_case) is commonly used in scripting languages like Python and as a constant in other C-styled languages.
- Pascal case (ex: PascalCase) is mainly reserved for class names, interfaces, and namespaces.
What’s next?
If you're setting up a new project or improving an existing one, I recommend you check out the series on enforcing conventions with Husky.
Additionally, I'm going to assume you're interested in learning about ways to improve the maintainability of your code. In that case, I recommend learning TDD, writing acceptance tests, and adopting a feature-driven approach to software design.
Stay in touch!
Join 20000+ value-creating Software Essentialists getting actionable advice on how to master what matters each week. 🖖
View more in Coding conventions